How to Blink LED using AT89C1051
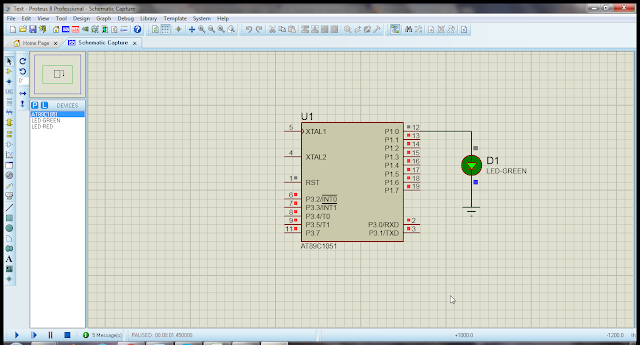
The following code demonstrate, how to blink LED using AT89C1051. The code is written in Keil uVision2 IDE and simulation is done with Proteus 8.0. At the end of code, you can find complete project files for download. Code Using Keil uVision2 #include // proto-types void Delayms(unsigned int x_time); // port pin labling sbit LED_0 = P1^0; void main(void) { // make pin as output LED_0 = 0; while(1) { LED_0 = ~LED_0; Delayms(500); } } void Delayms(unsigned int x_time) { unsigned int x,y; for(x=0;x<x_time;x++) for(y=0;y<122;y++); } Download Files For download Keil project and Proteus 8.0 simulation files, click here .