How to Program Interrupts in PIC16F877A
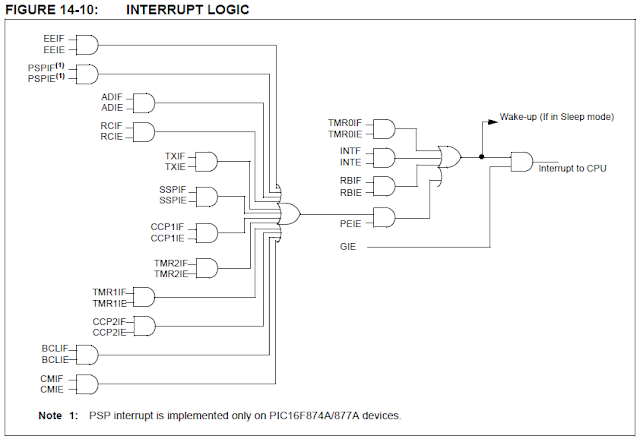
There are 15 interrupts available in PIC16F877A controller. I will try to demonstrate individual interrupt so that you can easily understand. The list is the following. 1. External Interrupt 2. RB Port Change Interrupt 3. Timer 0 Interrupt 4. Timer 1 Interrupt 5. Parallel Slave Port Read/Write Interrupt 6. A/D Converter Interrupt 7. USART Receive Interrupt 8. USART Transmit Interrupt 9. SPI (Slave) Interrupt 9. Synchronous Serial Port Interrupt 10. CCP1 (Capture, Compare, PWM) Interrupt 11. CCP2 (Capture, Compare, PWM) Interrupt 12. TMR2 to PR2 Match Interrupt 13. Comparator Interrupt 14. EEPROM Write Operation Interrupt 15. Bus Collision Interrupt 1. External Interrupt (RB0) The following code demonstrates how to use external interrupt (RB0). A push button is connected to RB0/INT, when push button is pressed a high to low signal is generated. As in option register external interrupt is configured on falling edge so interrupt is generated and interrupt serv